然后,浏览器是通过发送这个链接的HTTP要求,得到一个包含多个.ts视频片段地址的文件。浏览器会按顺序要求这些.ts文件,再用一个播放器来拼接和播放这些视频片段。但是有些网站会对m3u8链接进行隐蔽或者加密,使得无法直接从网页中找到它。这时候,你可以用开拓者工具的网络选项卡来监视浏览器发送的所有HTTP要求,看看有没有包含m3u8的要求。
有没有不该用浏览器的网络监视器得到m3u8链接呢?答案是有的,本文将先容如何通过浏览器扩展或注入代码的方法,来获取m3u8文件的链接。
详细来说,有以下几种可能的方法:
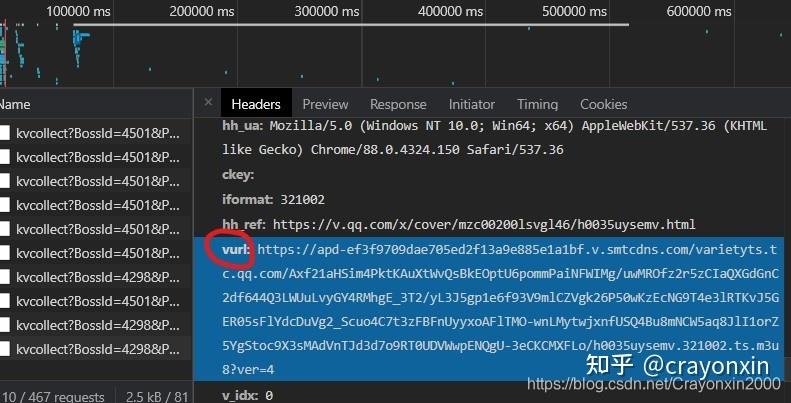
通过网络监视:浏览器插件可以利用chrome.webRequest API来监听浏览器发送或吸收的所有HTTP要求,然后过滤出包含m3u8的要求,并获取其url。这种方法的优点是大略直接,缺陷是可能会影响浏览器的性能和网络安全。
通过代码注入:浏览器插件可以利用chrome.tabs API或者chrome.extension API来向目标网页注入一段JavaScript代码,这段代码可以获取网页中的视频标签或者脚本代码,并从中提取出m3u8 url。这种方法的优点是可以更灵巧地适应不同的网页构造,缺陷是可能会毁坏网页的正常功能或者与其他插件冲突。
通过开拓者工具:浏览器插件可以利用chrome.devtools API来扩展浏览器的开拓者工具,添加一个专门用于获取m3u8 url的面板或者按钮。这种方法的优点是可以让用户更方便地操作,缺陷是须要用户主动打开开拓者工具并选择相应的功能。
网络监视:利用WinPcap或者Npcap等库来捕获网络数据包,然后过滤出包含m3u8的要求,并获取其url。以下是一个利用C#和SharpPcap库的示例代码:
using System;
using System.Text.RegularExpressions;
using SharpPcap;
using PacketDotNet;
namespace M3U8Sniffer
{
class Program
{
static void Main(string[] args)
{
// Get the list of available devices
var devices = CaptureDeviceList.Instance;
// Check if any device exists
if (devices.Count < 1)
{
Console.WriteLine("No device found.");
return;
}
// Print the list of devices
Console.WriteLine("The following devices are available:");
for (int i = 0; i < devices.Count; i++)
{
Console.WriteLine("{0}) {1}", i, devices[i].Description);
}
// Ask the user to choose a device
Console.WriteLine("Enter the device number (0-{0}):", devices.Count - 1);
int deviceIndex = int.Parse(Console.ReadLine());
// Get the chosen device
var device = devices[deviceIndex];
// Open the device with default configuration
device.Open();
// Set a filter to capture only HTTP requests
device.Filter = "tcp port 80";
// Register a handler for packet arrival event
device.OnPacketArrival += new PacketArrivalEventHandler(OnPacketArrival);
// Start capturing packets
Console.WriteLine("Start capturing on {0}", device.Description);
device.StartCapture();
// Wait for 'Enter' from the user.
Console.WriteLine("Press Enter to stop...");
Console.ReadLine();
// Stop capturing packets
device.StopCapture();
// Close the device
device.Close();
}
// The handler for packet arrival event
private static void OnPacketArrival(object sender, CaptureEventArgs e)
{
// Parse the packet as TCP packet
var packet = Packet.ParsePacket(e.Packet.LinkLayerType, e.Packet.Data);
var tcpPacket = packet.Extract<TcpPacket>();
// Check if the TCP packet contains payload
if (tcpPacket != null && tcpPacket.PayloadData != null)
{
// Convert the payload data to string
var data = System.Text.Encoding.UTF8.GetString(tcpPacket.PayloadData);
// Check if the data is an HTTP request
if (data.StartsWith("GET") || data.StartsWith("POST"))
{
// Extract the request URI from the data
var lines = data.Split('\n');
var uri = lines[0].Split(' ')[1];
// Check if the URI contains m3u8
if (uri.Contains("m3u8"))
{
// Print the m3u8 url to the console
Console.WriteLine("Found m3u8 url: {0}", uri);
}
}
}
}
}
}
代码注入(js代码注入):利用chrome.tabs API或者chrome.extension API来向目标网页注入一段JavaScript代码,这段代码可以获取网页中的视频标签或者脚本代码,并从中提取出m3u8 url。以下是一个利用JavaScript和chrome.tabs API的示例代码:
// Get the current active tab in the current window
chrome.tabs.query({active: true, currentWindow: true}, function(tabs) {
// Get the first tab object in the array
var tab = tabs[0];
// Inject a content script into the tab
chrome.tabs.executeScript(tab.id, {
code: `
// Get all the video elements in the document
var videos = document.getElementsByTagName('video');
// Loop through each video element
for (var i = 0; i < videos.length; i++) {
// Get the video source attribute or source child element
var src = videos[i].src || videos[i].querySelector('source').src;
// Check if the source contains m3u8
if (src.includes('m3u8')) {
// Send the m3u8 url to the background script
chrome.runtime.sendMessage({url: src});
}
}
// Get all the script elements in the document
var scripts = document.getElementsByTagName('script');
// Create a regular expression to match m3u8 url
var regex = /https?:\/\/\S+\.m3u8\b/g;
// Loop through each script element
for (var i = 0; i < scripts.length; i++) {
// Get the script text content
var text = scripts[i].textContent;
// Check if the text matches the regex
var match = regex.exec(text);
if (match) {
// Send the m3u8 url to the background script
chrome.runtime.sendMessage({url: match[0]});
}
}
`
});
});
// Listen for messages from the content script
chrome.runtime.onMessage.addListener(function(message, sender, sendResponse) {
// Check if the message contains a url
if (message.url) {
// Print the m3u8 url to the console
console.log("Found m3u8 url: " + message.url);
}
});
代码注入(dll代码注入):利用DLL注入等技能来修正目标进程的内存,然后实行一段代码来获取m3u8 url。以下是一个利用C++和Windows API的示例代码:
#include <windows.h>
#include <tlhelp32.h>
#include <iostream>
// A function to get the process id by name
DWORD GetProcessIdByName(const char name)
{
// Create a snapshot of all processes
HANDLE snapshot = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
// Check if the snapshot is valid
if (snapshot != INVALID_HANDLE_VALUE)
{
// Initialize a PROCESSENTRY32 structure
PROCESSENTRY32 entry;
entry.dwSize = sizeof(PROCESSENTRY32);
// Get the first process in the snapshot
if (Process32First(snapshot, &entry))
{
// Loop through all processes in the snapshot
do
{
// Compare the process name with the given name
if (strcmp(entry.szExeFile, name) == 0)
{
// Return the process id
return entry.th32ProcessID;
}
} while (Process32Next(snapshot, &entry));
}
}
// Return 0 if not found or failed
return 0;
}
// A function to inject a DLL into a process by id
BOOL InjectDll(DWORD pid, const char dllPath)
{
// Open the target process with all access
HANDLE process = OpenProcess(PROCESS_ALL_ACCESS, FALSE, pid);
// Check if the process handle is valid
if (process != NULL)
{
// Get the address of LoadLibraryA function in kernel32.dll module
LPVOID loadLibraryAddr = GetProcAddress(GetModuleHandleA("kernel32.dll"), "LoadLibraryA");
// Check if the address is valid
if (loadLibraryAddr != NULL)
{
// Allocate memory in the target process for the DLL path
LPVOID dllPathAddr = VirtualAllocEx(process, NULL, strlen(dllPath) + 1, MEM_COMMIT, PAGE_READWRITE);
// Check if the memory allocation is successful
if (dllPathAddr != NULL)
{
// Write the DLL path to the allocated memory
BOOL writeResult = WriteProcessMemory(process, dllPathAddr, dllPath, strlen(dllPath) + 1, NULL);
// Check if the write operation is successful
if (writeResult)
{
// Create a remote thread in the target process to execute LoadLibraryA with DLL path as argument
HANDLE thread = CreateRemoteThread(process, NULL, 0, (LPTHREAD_START_ROUTINE)loadLibraryAddr, dllPathAddr, 0, NULL);
// Check if the thread handle is valid
if (thread != NULL)
{
// Wait for the thread to finish
WaitForSingleObject(thread, INFINITE);
// Close the thread handle
CloseHandle(thread);
// Free the allocated memory in the target process
VirtualFreeEx(process, dllPathAddr, 0, MEM_RELEASE);
// Close the process handle
CloseHandle(process);
// Return true as success
return true;
}
}
// Free the allocated memory in the target process
VirtualFreeEx(process, dllPathAddr, 0, MEM_RELEASE);
}
}
// Close the process handle
CloseHandle(process);
}
开拓者工具的代码:利用chrome.devtools API来扩展浏览器的开拓者工具,添加一个专门用于获取m3u8 url的面板或者按钮。以下是一个利用JavaScript和chrome.devtools API的示例代码:
// Define a panel in the devtools
chrome.devtools.panels.create("M3U8 Sniffer", "icon.png", "panel.html", function(panel) {
// Register a callback for panel shown event
panel.onShown.addListener(function(window) {
// Get the current inspected tab id
var tabId = chrome.devtools.inspectedWindow.tabId;
// Send a message to the background script to start capturing packets
chrome.runtime.sendMessage({action: "start", tabId: tabId});
// Listen for messages from the background script
chrome.runtime.onMessage.addListener(function(message, sender, sendResponse) {
// Check if the message contains a url and matches the tab id
if (message.url && message.tabId == tabId) {
// Append the m3u8 url to the panel window
window.document.body.innerHTML += "<p>" + message.url + "</p>";
}
});
});
// Register a callback for panel hidden event
panel.onHidden.addListener(function(window) {
// Get the current inspected tab id
var tabId = chrome.devtools.inspectedWindow.tabId;
// Send a message to the background script to stop capturing packets
chrome.runtime.sendMessage({action: "stop", tabId: tabId});
});
});
这些代码编译后,如何安装到浏览器中呢?请对4种方法分别解释一下安装过程:
网络监视:这种方法须要编译成一个可实行文件,然后运行在后台,不须要安装到浏览器中。但是须要用户自己启动和关闭程序,并且可能须要管理员权限。
代码注入(js代码注入):这种方法须要编译成一个浏览器扩展,然后在浏览器的扩展管理页面进行安装。详细的步骤可以参考这个链接:https://developer.chrome.com/docs/extensions/mv3/getstarted/
代码注入(dll代码注入):这种方法须要编译成一个DLL文件,然后利用一些工具或者程序来将其注入到目标进程中。详细的步骤可以参考这个链接:https://www.codeproject.com/Articles/2082/API-hooking-revealed
开拓者工具:这种方法也须要编译成一个浏览器扩展,然后在浏览器的扩展管理页面进行安装。详细的步骤可以参考这个链接:https://developer.chrome.com/docs/extensions/mv3/devtools/
如果要问哪种方法最方便最安全?
这个问题的答案可能比较主不雅观,不同的人可能有不同的意见。我的意见是:
我以为开拓者工具的方法最方便最安全,由于它只须要安装一个浏览器扩展,然后在须要的时候打开开拓者工具就可以利用。它不会影响浏览器的性能和安全,也不会修正目标进程的内存。
我以为代码注入(js代码注入)的方法最方便最安全,由于它可以适应不同的网页构造,而且只须要注入一段JavaScript代码,不会对目标进程造成太大的影响。它也只须要安装一个浏览器扩展,而且可以自动运行。
我以为网络监视的方法最方便最安全,由于它可以捕获所有的HTTP要求,而不须要关心网页的详细内容。它也不须要安装任何浏览器扩展,只须要运行一个可实行文件就可以了。它也不会修正目标进程的内存,只是读取网络数据包。
我以为代码注入(dll代码注入)的方法最方便最安全,由于它可以直接在目标进程中实行任意代码,而不受浏览器的限定。它也不须要安装任何浏览器扩展,只须要利用一些工具或者程序就可以了。它也可以实现更多的功能,而不但是获取m3u8链接。
什么是浏览器扩展?
浏览器扩展是一种可以增强浏览器功能和用户体验的软件组件。它们可以在浏览器中运行一些代码,访问一些API,修正一些网页内容,或者供应一些额外的界面和功能。举例如下:
可以增强浏览器的历史记录功能,让用户可以按照日期查看浏览过的网页1
可以增强浏览器的拼写和语法检讨功能,让用户在写作时可以自动纠正缺点2
可以增强浏览器的截图和标注功能,让用户可以方便地截取和编辑网页内容1
可以增强浏览器的阅读体验,让用户可以在一个干净和沉浸式的界面中阅读网页内容3
可以增强浏览器的安全性和隐私性,让用户可以拦截广告和恶意网站,或者利用 VPN 代理来保护自己的网络活动1
浏览器扩展可以访问一些特定的 API,来实现一些分外的功能。以下是一些例子:
可以访问 chrome.devtools API 来扩展浏览器的开拓者工具,添加一些专门用于获取 m3u8 url 的面板或者按钮4
可以访问 chrome.tabs API 来管理浏览器的标签页,实现一些暂存和规复标签页的功能
可以访问 chrome.storage API 来存储和同步扩展的数据,实现一些书签和条记的功能
可以访问 chrome.webRequest API 来监视和修正网络要求,实现一些网络剖析和过滤的功能
浏览器扩展可以供应额外的界面和功能,来增加用户的便利性和意见意义性。以下是一些例子:
可以供应一个新标签页,来展示一些俏丽的背景图片和诗词
可以供应一个侧边栏,来显示一些实用的工具和信息
可以供应一个悬浮窗口,来显示一些翻译或者定义
可以供应一个游戏界面,来让用户在新标签页中玩一些小游戏
浏览器扩展可以修正网页内容,来实现一些个性化或者优化的目的。以下是一些例子:
可以修正网页的字体或者颜色,来适应用户的喜好或者视力需求
可以修正网页的布局或者样式,来适应用户的屏幕大小或者设备类型
可以修正网页的内容或者元素,来去除一些不须要或者滋扰的部分
可以修正网页的脚本或者事宜,来增加一些交互或者动画效果
如何开拓浏览器扩展?
要开拓一个浏览器扩展,常日须要以下几个步骤:
首先,你须要创建一个 manifest.json 文件,来声明你的扩展的基本信息,如名称,版本,描述,图标,权限等12。
然后,你须要创建一些 JavaScript 文件,来定义你的扩展的功能和逻辑。你可以利用一些特定的 API,来与浏览器或者网页进行交互123。
接着,你须要创建一些 HTML 和 CSS 文件,来定义你的扩展的用户界面和样式。你可以利用一些特定的元素,来创建弹出窗口,选项页面,内容脚本等124。
末了,你须要在浏览器中加载和测试你的扩展。你可以在浏览器的扩展管理页面中启用开拓者模式,然后选择你的扩展所在的文件夹34。你可以在浏览器中查看你的扩展的效果和缺点信息。
详细步骤如下:
创建一个 manifest.json 文件,来声明我们的扩展的基本信息,如名称,版本,描述,图标,权限等。例如:
{
"manifest_version": 2,
"name": "M3U8 Finder",
"version": "1.0",
"description": "A simple extension that finds all m3u8 links in the current web page.",
"browser_action": {
"default_icon": "icon.png",
"default_popup": "popup.html"
},
"permissions": [
"tabs"
]
}
然后,我们须要创建一个 icon.png 文件,来作为我们的扩展的图标。这个文件可以是任意的图片文件,但是最好是 16x16 或者 32x32 的尺寸。例如:
;
// 定义一个函数,用来格式化韶光
function formatTime(date) {
var hours = date.getHours();
var minutes = date.getMinutes();
var seconds = date.getSeconds();
// 补零
if (hours < 10) {
hours = "0" + hours;
}
if (minutes < 10) {
minutes = "0" + minutes;
}
if (seconds < 10) {
seconds = "0" + seconds;
}
// 返回格式化后的韶光字符串
return hours + ":" + minutes + ":" + seconds;
}
// 定义一个函数,用来更新韶光
function updateTime() {
// 获取当前韶光
var now = new Date();
// 格式化韶光
var timeString = formatTime(now);
// 显示韶光
timeElement.textContent = timeString;
}
// 调用一次更新韶光函数,以显示初始韶光
updateTime();
// 设置一个定时器,每隔一秒更新一次韶光
setInterval(updateTime, 1000);
以上便是一个大略的浏览器扩展的例子。你可以把这些文件放在同一个文件夹里,然后在浏览器中加载和测试它。