Id 文章id
Title 文章标题
Content 文章内容
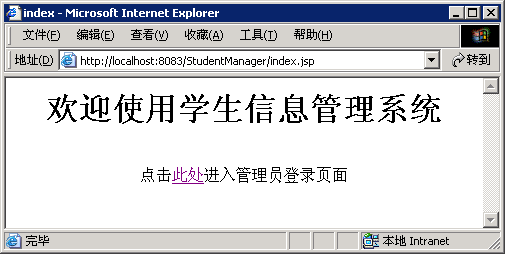
Category_id 文章分类id
Dateline 韶光
set names gbk;
create database cms character set utf8;
use cms;
create table article (
id int primary key auto_increment,#文章id
title varchar(30),#文章标题
content varchar(20),#文章内容
category_id int,#文章分类ID
datetime date#韶光
);
2、写一个操作mysql的类,类名为mysqlDB,类中有连接数据库方法connect()和返回数据库查询结果的二维数组的方法getAll();
mysqlDB.class.php
<?php
//数据库封装类
classmysqlDB
{
public$conn = NULL;//连接工具
public$rs = NULL;//结果集工具
//连接数据库
private function__construct()
{
$this->conn = mysql_connect(\"大众localhost\公众,\公众name\"大众,\"大众password\"大众);
mysql_query(\"大众set names utf8\"大众);
mysql_select_db(\"大众database\公众);
}
//防止克隆工具
private function__clone()
{}
//得到该类的工具(单例模式的)
public static functiongetInstance()
{
static$obj = NULL;
if($obj == NULL)
{
$obj = newmysqlDB();
}
return$obj;
}
//实行select语句,返回:二维数组
public functiongetAll($sql)
{
$result = array();//存储所有记录
$this->rs = mysql_query($sql);
while($row = mysql_fetch_array($this->rs))
{
$result[] = $row;
}
return$result;
}
//实行select语句,返回:一维关联数组
public functiongetRow($sql)
{
$result = NULL;//存储一条记录
$this->rs = mysql_query($sql);
if($row = mysql_fetch_array($this->rs))
{
$result = $row;
}
return$result;
}
//实行insert、update、delete语句,返回:受影响的行数
public functionexec($sql)
{
mysql_query($sql);
$result = mysql_affected_rows($this->conn);
return$result;
}
//开释结果集
public functionfreeResult()
{
mysql_free_result($this->rs);
}
//关闭数据库
public functionclose()
{
mysql_close($this->conn);
}
}
?>
3、用第一题中的表和第二题中的类写出如下操作:显示点击量最多的10条新闻的标题和发布韶光。
insert into article values(' ','标题1','内容1','文章分类ID1',now());
insert into article values(' ','标题2','内容2','文章分类ID2',now());
insert into article values(' ','标题3','内容3','文章分类ID3',now());
insert into article values(' ','标题4','内容4','文章分类ID4',now());
insert into article values(' ','标题5','内容5','文章分类ID5',now());
insert into article values(' ','标题6','内容6','文章分类ID6',now());
insert into article values(' ','标题7','内容7','文章分类ID7',now());
insert into article values(' ','标题8','内容8','文章分类ID8',now());
insert into article values(' ','标题9','内容9','文章分类ID9',now());
insert into article values(' ','标题10','内容10','文章分类ID10',now());
insert into article values(' ','标题11','内容11','文章分类ID11',now());
insert into article values(' ','标题12','内容12','文章分类ID12',now());
insert into article values(' ','标题13','内容13','文章分类ID13',now());
select from article;
<?php
header(\"大众content-type:text/html;charset=utf-8\公众);
include_once'mysqlDB.class.php';
$sql = \公众select title,datetime from article limit 10\"大众;
$conn = mysqlDB::getInstance();
$result = $conn->getAll($sql);
?>
<!DOCTYPE html PUBLIC \公众-//W3C//DTD HTML 4.01 Transitional//EN\"大众 \"大众http://www.w3.org/TR/html4/loose.dtd\"大众>
<html>
<head>
<meta http-equiv=\公众Content-Type\"大众 content=\"大众text/html; charset=UTF-8\"大众>
<title>Insert title here</title>
</head>
<body>
<table border='1' align=\"大众center\"大众>
<tr>
<td>标题</td>
<td>韶光</td>
</tr>
<?php
foreach($result as$v){
?>
<tr>
<td><?php echo$v['title']?></td>
<td><?php echo$v['datetime']?></td>
</tr>
<?php
}
?>
</table>
</body>
</html>
4、如果要把article表分成多个表,写出你的大体思路。
create table table2 select from table1 order by id limit 1,100
create table table3 select from table1 order by id limit 100,100
create table table4 select from table1 order by id limit 200,100
5、请写一个函数验证电子邮件的格式是否精确。
[\w!#$%&'+/=?^_`{|}~-]+(?:\.[\w!#$%&'+/=?^_`{|}~-]+)@(?:[\w](?:[\w-][\w])?\.)+[\w](?:[\w-][\w])?